In distributed systems, keeping data consistent across multiple devices or servers is a significant challenge. CRDTs offer an innovative solution to this problem, changing how we manage data in distributed environments. This article explores CRDTs, their inner workings, and their growing importance in modern technology.
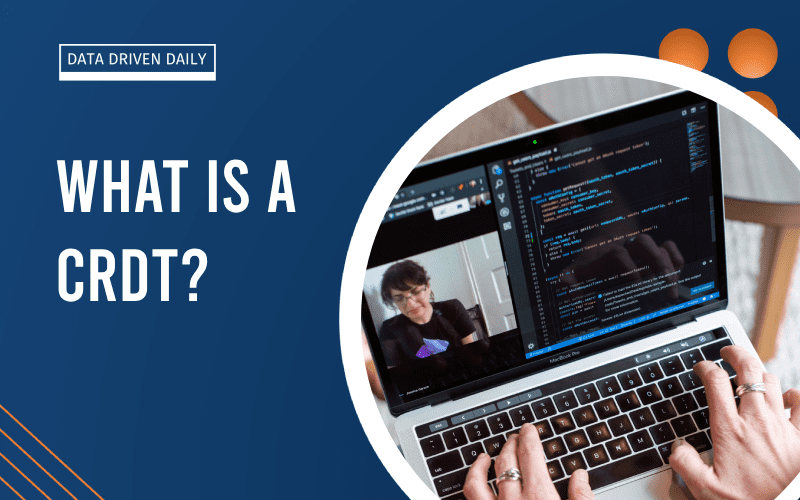
Understanding CRDTs: The Basics
CRDT stands for Conflict-free Replicated Data Type. It’s a special kind of data structure designed to be replicated across multiple computers in a network, allowing each replica to be updated independently and concurrently without coordination between the replicas, and still achieve consistency.
But what does that mean in plain English? Let’s break it down:
- Conflict-free: CRDTs are designed to automatically resolve conflicts that might arise when multiple users or devices make changes to the same data simultaneously.
- Replicated: The data is copied and stored on multiple devices or servers.
- Data Type: CRDTs are specific data structures, like counters, sets, or text, with special properties that make them suitable for distributed systems.
How CRDTs Work: The Magic Behind the Scenes
CRDTs work on a principle that might seem counterintuitive at first: instead of trying to prevent conflicts, they embrace them. Here’s how:
- Local Updates: Each replica can be updated independently without immediate synchronization with other replicas.
- Eventual Consistency: Over time, all replicas will converge to the same state, even if they received updates in different orders.
- Monotonic Progress: Once information is added to a CRDT, it’s never “undone” – the data only grows or evolves in a forward direction.
- Commutative Operations: The order of operations doesn’t matter – you’ll get the same result regardless of the sequence of updates.
Let’s look at a simple example:
# A simple counter CRDT
class CounterCRDT:
def __init__(self):
self.value = 0
def increment(self):
self.value += 1
def merge(self, other):
self.value = max(self.value, other.value)
# Usage
counter1 = CounterCRDT()
counter2 = CounterCRDT()
counter1.increment() # counter1 = 1
counter2.increment() # counter2 = 1
counter2.increment() # counter2 = 2
counter1.merge(counter2) # counter1 = 2
counter2.merge(counter1) # counter2 = 2
In this example, both counters end up with the same value, regardless of the order of operations or when they synced.
Types of CRDTs: A Toolkit for Distributed Data
CRDTs come in various flavors, each designed for specific use cases:
- Counter CRDTs: For keeping track of numerical values that can be incremented or decremented.
- Set CRDTs: For managing collections of unique items.
- Text CRDTs: For collaborative text editing, like in Google Docs.
- Map CRDTs: For key-value pairs that can be updated concurrently.
- Graph CRDTs: For maintaining distributed graph structures.
Here’s a quick comparison of some common CRDT types:
CRDT Type | Use Case | Example Operation |
---|---|---|
Counter | Analytics | Increment view count |
Set | Todo lists | Add/remove items |
Text | Collaborative editing | Insert/delete characters |
Map | User profiles | Update user properties |
Graph | Social networks | Add/remove connections |
Real-World Applications: CRDTs in Action
CRDTs are more than just a cool concept – they’re solving real problems in various industries:
- Collaborative Software: Tools like Google Docs use CRDTs to allow multiple users to edit documents simultaneously without conflicts.
- Distributed Databases: Databases like Riak and AntidoteDB use CRDTs to maintain consistency across distributed systems.
- Offline-First Mobile Apps: CRDTs enable apps to work offline and sync seamlessly when a connection is restored.
- Multiplayer Games: Game state can be managed using CRDTs to ensure consistency across players’ devices.
- IoT Systems: CRDTs help manage data from multiple sensors and devices that may have intermittent connectivity.
CRDTs vs. Traditional Consensus Algorithms
While CRDTs provide a unique approach to data consistency, it’s important to understand how they compare to traditional consensus algorithms like Paxos or Raft. Let’s break down the key differences:
- Coordination Requirements:
- Traditional algorithms: Require explicit coordination between nodes.
- CRDTs: Operate without direct coordination, allowing for independent updates.
- Network Dependency:
- Traditional algorithms: Often struggle with network partitions or high latency.
- CRDTs: Can continue functioning even with unreliable network conditions.
- Consistency Model:
- Traditional algorithms: Typically provide strong consistency.
- CRDDs: Offer eventual consistency, with potential temporary divergence between replicas.
- Scalability:
- Traditional algorithms: May face scalability issues with a large number of nodes.
- CRDTs: Generally scale well, even with many replicas.
- Use Cases:
- Traditional algorithms: Suited for scenarios requiring immediate strong consistency.
- CRDTs: Ideal for applications that can tolerate eventual consistency for better availability.
Here’s a simple comparison table:
Aspect | Traditional Consensus | CRDTs |
---|---|---|
Coordination | Required | Not required |
Network Resilience | Low | High |
Consistency | Strong | Eventual |
Scalability | Limited | High |
Latency | Higher | Lower |
Understanding these differences can help you choose the right approach for your distributed system needs.
CRDT Design Patterns and Best Practices
When working with CRDTs, certain design patterns and best practices can help you create more efficient and maintainable systems:
- Delta CRDTs:
- Instead of sending the entire CRDT state, only send the changes (deltas) between synchronizations.
- This can significantly reduce network traffic and improve performance.
- Hybrid CRDT Approaches:
- Combine different CRDT types to model complex data structures.
- For example, use a set CRDT to manage document IDs and a separate text CRDT for each document’s content.
- Operational Transformation Compatibility:
- Some systems combine CRDTs with Operational Transformation (OT) for text editing.
- This can provide the benefits of both approaches in collaborative editing scenarios.
- State-based vs. Operation-based CRDTs:
- State-based CRDTs synchronize by merging entire states.
- Operation-based CRDTs synchronize by sharing operations.
- Choose based on your network constraints and data size.
- Conflict Resolution Strategies:
- Implement application-specific conflict resolution when the default CRDT behavior isn’t sufficient.
- For example, in a shared calendar, you might need custom logic to handle overlapping event creations.
- Versioning and Pruning:
- Implement versioning to track the evolution of your CRDT data.
- Use pruning techniques to remove obsolete data and prevent unbounded growth.
Here’s a simple example of a delta CRDT approach in Python:
class DeltaCounterCRDT:
def __init__(self):
self.value = 0
self.delta = 0
def increment(self, amount=1):
self.value += amount
self.delta += amount
def get_and_reset_delta(self):
delta = self.delta
self.delta = 0
return delta
def apply_delta(self, delta):
self.value += delta
# Usage
counter = DeltaCounterCRDT()
counter.increment(5)
delta = counter.get_and_reset_delta() # delta = 5, counter.delta = 0
# Send delta to other replicas
counter.apply_delta(3) # Simulating receiving a delta from another replica
This approach allows for more efficient synchronization, especially for counters with frequent updates.
CRDTs in Peer-to-Peer Systems
Peer-to-peer (P2P) systems present unique challenges for data consistency and synchronization. CRDTs are particularly well-suited for P2P environments due to their decentralized nature. Let’s explore how CRDTs can be applied in P2P systems:
- Decentralized File Sharing:
- Use set CRDTs to manage file metadata across peers.
- Employ vector clocks with CRDTs to track file versions.
- Distributed Social Networks:
- Manage user profiles with map CRDTs.
- Handle friend connections using graph CRDTs.
- P2P Messaging Systems:
- Use CRDTs to ensure message ordering and delivery in group chats.
- Implement read receipts with counter CRDTs.
- Distributed Hash Tables (DHTs):
- Enhance DHTs with CRDTs to manage node joins and departures.
- Use CRDTs to replicate key-value pairs across multiple nodes.
- Blockchain and Cryptocurrency:
- Implement CRDT-based sidechains for improved scalability.
- Use CRDTs to manage off-chain state in layer-2 solutions.
Challenges in P2P CRDT implementations:
- Network Churn: P2P networks often have nodes joining and leaving frequently. CRDTs need to handle this churn gracefully.
- Limited Resources: Some P2P nodes (like mobile devices) may have limited storage or processing power, requiring efficient CRDT designs.
- Security and Trust: In open P2P networks, CRDTs need to be designed with security in mind to prevent malicious nodes from corrupting data.
- Network Partitions: P2P networks are prone to partitions. CRDTs should continue functioning and reconcile efficiently when partitions heal.
Here’s a basic example of how you might use a CRDT in a P2P file-sharing scenario:
import time
class FileMetadataCRDT:
def __init__(self, file_id):
self.file_id = file_id
self.versions = {} # Peer ID -> (timestamp, file_hash)
def update(self, peer_id, file_hash):
timestamp = time.time()
if peer_id not in self.versions or timestamp > self.versions[peer_id][0]:
self.versions[peer_id] = (timestamp, file_hash)
def merge(self, other):
for peer_id, (timestamp, file_hash) in other.versions.items():
if peer_id not in self.versions or timestamp > self.versions[peer_id][0]:
self.versions[peer_id] = (timestamp, file_hash)
def get_latest_version(self):
if not self.versions:
return None
return max(self.versions.items(), key=lambda x: x[1][0])
# Usage in a P2P network
file_metadata = FileMetadataCRDT("file123")
file_metadata.update("peer1", "hash1")
file_metadata.update("peer2", "hash2")
# Simulate receiving updates from another peer
other_metadata = FileMetadataCRDT("file123")
other_metadata.update("peer3", "hash3")
file_metadata.merge(other_metadata)
latest = file_metadata.get_latest_version()
print(f"Latest version: {latest}")
This example shows how file metadata can be managed across multiple peers, allowing for updates and merges while maintaining a consistent view of the latest file version.
By leveraging CRDTs in P2P systems, developers can create robust, decentralized applications that maintain consistency even in challenging network conditions.
Advantages of CRDTs: Why They’re Game-Changers
CRDTs offer several key benefits that make them attractive for modern distributed systems:
- Improved Availability: Systems can continue to function and accept updates even when network connections are unreliable.
- Reduced Latency: Local updates can be applied immediately without waiting for network round-trips.
- Scalability: CRDTs can handle large numbers of replicas and frequent updates.
- Simplicity: They provide a straightforward way to handle complex distributed data scenarios.
- Conflict Resolution: Automatic conflict resolution reduces the need for manual intervention.
Challenges and Limitations: Nothing’s Perfect
While CRDTs are powerful, they’re not a silver bullet. Here are some challenges to consider:
- Data Size: Some CRDTs can grow large over time, especially those that keep a history of all operations.
- Complexity: Implementing CRDTs correctly can be tricky, especially for more complex data types.
- Limited Operations: Not all operations can be easily expressed as CRDTs.
- Performance Overhead: The conflict resolution mechanisms can introduce some overhead.
- Eventual Consistency: While CRDTs guarantee eventual consistency, they may not provide strong consistency immediately after updates.
Implementing CRDTs: Getting Your Hands Dirty
If you’re interested in implementing CRDTs in your projects, here are some tips to get started:
- Choose the Right CRDT: Select a CRDT type that fits your data model and use case.
- Use Existing Libraries: Consider using established CRDT libraries like Automerge or Yjs instead of implementing from scratch.
- Test Thoroughly: Simulate concurrent updates and network partitions to ensure your CRDT behaves correctly.
- Consider Performance: Think about data growth and synchronization costs, especially for large datasets.
- Implement Garbage Collection: For long-lived systems, implement ways to prune old data to keep CRDT sizes manageable.
Here’s a simple example of a grow-only set CRDT in Python:
class GSetCRDT:
def __init__(self):
self.elements = set()
def add(self, element):
self.elements.add(element)
def merge(self, other):
self.elements = self.elements.union(other.elements)
def __contains__(self, element):
return element in self.elements
# Usage
set1 = GSetCRDT()
set2 = GSetCRDT()
set1.add("apple")
set2.add("banana")
set1.merge(set2)
print("apple" in set1) # True
print("banana" in set1) # True
The Future of CRDTs: What’s Next?
As distributed systems continue to grow in importance, CRDTs are likely to play an increasingly significant role. Here are some trends to watch:
- Integration with Blockchain: CRDTs could be used to improve the scalability and performance of blockchain systems.
- Edge Computing: As computing moves closer to the edge, CRDTs can help manage data across widely distributed nodes.
- AI and Machine Learning: CRDTs might be used to manage distributed training data or model parameters in federated learning scenarios.
- Standardization: We may see efforts to standardize CRDT implementations and protocols.
- New CRDT Types: Researchers are continually developing new types of CRDTs for more complex data structures and use cases.
Wrapping Up: The Power of Conflict-Free Replication
CRDTs represent a powerful approach to managing data in distributed systems. By embracing the inherent challenges of distributed computing rather than fighting against them, CRDTs offer a way to build more resilient, scalable, and responsive systems.
Whether you’re building collaborative software, designing distributed databases, or working on the next big thing in edge computing, understanding CRDTs can give you a valuable tool in your distributed systems toolkit.
As with any technology, it’s important to weigh the pros and cons and consider whether CRDTs are the right fit for your specific use case. But with their growing adoption and continuous development, CRDTs are certainly a technology worth keeping an eye on in the ever-evolving landscape of distributed systems.
Ben is a full-time data leadership professional and a part-time blogger.
When he’s not writing articles for Data Driven Daily, Ben is a Head of Data Strategy at a large financial institution.
He has over 14 years’ experience in Banking and Financial Services, during which he has led large data engineering and business intelligence teams, managed cloud migration programs, and spearheaded regulatory change initiatives.